Bash scripting is one of the most popular, accessible ways of programming your Linux computer. These simple script examples will help you understand the process and introduce you to the basics of Bash programming.
1. How to Print Hello World in Bash
The Hello World example is a great way of learning about any programming language, and Bash is no exception.
Here’s how to print “Hello World” using Bash:
- Open a text editor and start editing a new file containing the following lines of code.
- The first line of your Bash scripts should always look like this:
#!/bin/bash
The Shebang command (#!/bin/bash) is essential as the shell uses it to decide how to run the script. In this case, it uses the Bash interpreter. - Any line starting with a # symbol is a comment. The shebang line is a special case, but you can use your own comments to explain your code. Add a comment on line 2, e.g.:
# Print some text from a bash script
- You can print to standard output using the echo command, followed by the value you want to print. Add the following on line 3:
echo "Hello World"
- Save the script, preferably with a .sh extension, e.g. hello_world.sh. The extension is not a requirement, but it’s a convention that is useful to stick to.
- To run your script, make the file executable. Use the chmod (“change mode”) command together with the +x (“executable”) argument and the name of your shell script:
chmod +x hello_world.sh
- Use this command to run the script from within its directory:
./hello_world.sh
- When the script runs, it will print the text “Hello World” to your terminal:
2. Create a Directory by Reading Input
From your scripts, you can run any program that you might normally run on the command line. For example, you can create a new directory from your script using the mkdir command.
- Begin with the same shebang line as before:
#!/bin/bash
- Prompt the user for a directory name, using the echo command as before:
echo "Enter new directory name:"
- Use the built-in read command to fetch user input. The single argument names a variable that the shell will store the input in:
read newdir
- When you need to use the value stored in a variable, prefix its name with a dollar symbol ($). You can pass the contents of the input variable as an argument to the mkdir command, to create a new directory:
mkdir $newdir
- When you run this script, it will prompt you for input. Enter a valid directory name and you’ll see that the script creates it in your current directory:
3. Create a Directory Using Command Line Arguments
As an alternative to reading input interactively, most Linux commands support arguments. You can supply an argument when you run a program, to control its behavior.
Within your script, you can use $1 to refer to a special variable that contains the value of the first argument. $2 will refer to the second argument, and so on.
- Create a directory using the mkdir command from the previous example. However, this time, use the built-in variable $1:
#!/bin/bash
mkdir $1 - Run the script, this time passing your chosen name of a new directory as an argument:
./arg_dir.sh Test
You may be wondering what happens if you run the script without supplying an argument at all. Try it and see; you should receive an error message that starts “usage: mkdir”:
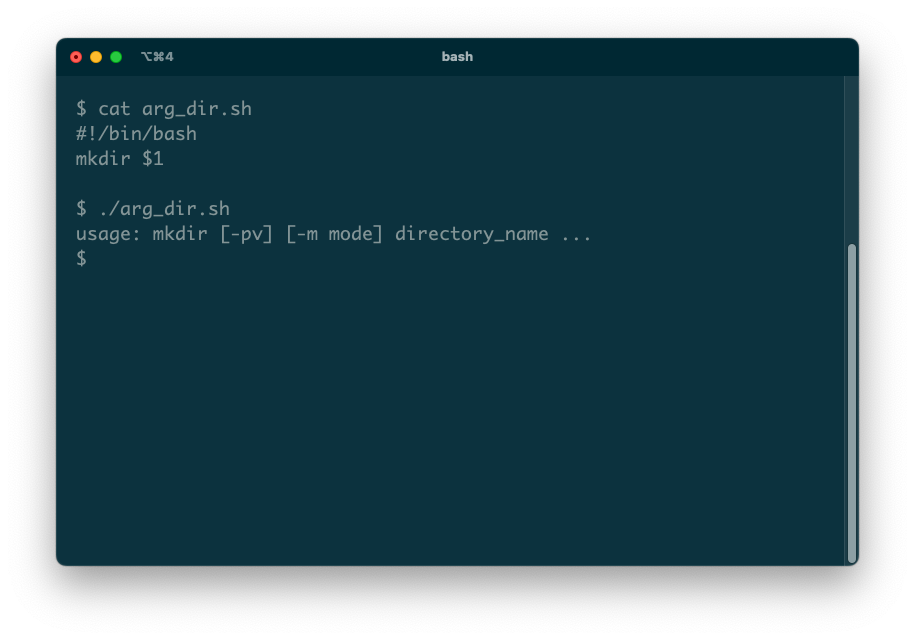
Without any command-line arguments, the value of $1 will be empty. When your script calls mkdir, it won’t be passing an argument to it, and the mkdir command will return that error. To avoid this, you can check for the condition yourself and present a more friendly error:
- As always, begin with the shebang line:
#!/bin/bash
- Before you call mkdir, check for an empty first argument (i.e. no arguments). You can do this using Bash’s if statement which runs code based on a condition:
if ["$1" = ""]; then
- If the first argument is empty, print an error and exit your script:
echo "Please provide a new directory name as the first argument"
exit - The slightly strange “fi” keyword (“if” reversed) signals the end of an if statement in Bash:
fi
- Your script can now continue as before, to handle the case when an argument is present:
mkdir $1
When you run this new version of the script, you’ll get a message if you forget to include an argument:
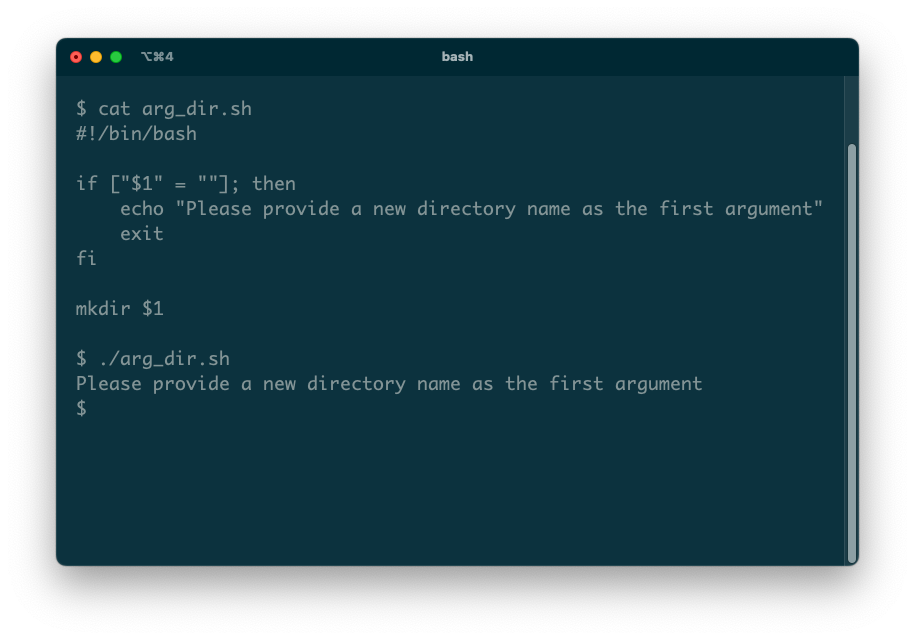
4. Delete a File Using a Bash Function
If you find yourself repeating the same code, consider wrapping it in a function. You can then call that function whenever you need to.
Here’s an example of a function that deletes a given file.
- Start with the shebang line:
#!/bin/bash
- Define a function by writing its name followed by empty parentheses and commands inside curly brackets:
del_file() {
You can then call the function and pass it the name of a file to delete:
echo "deleting $1"
rm $1
}del_file test.txt
When you call a function, it will set the special $? value with the exit status of the last command it runs. The exit status is useful for error checking; in this example, you can test whether the rm command succeeded:
if [ $? -ne 0 ]; then
echo "Sorry, could not delete the file"
fi
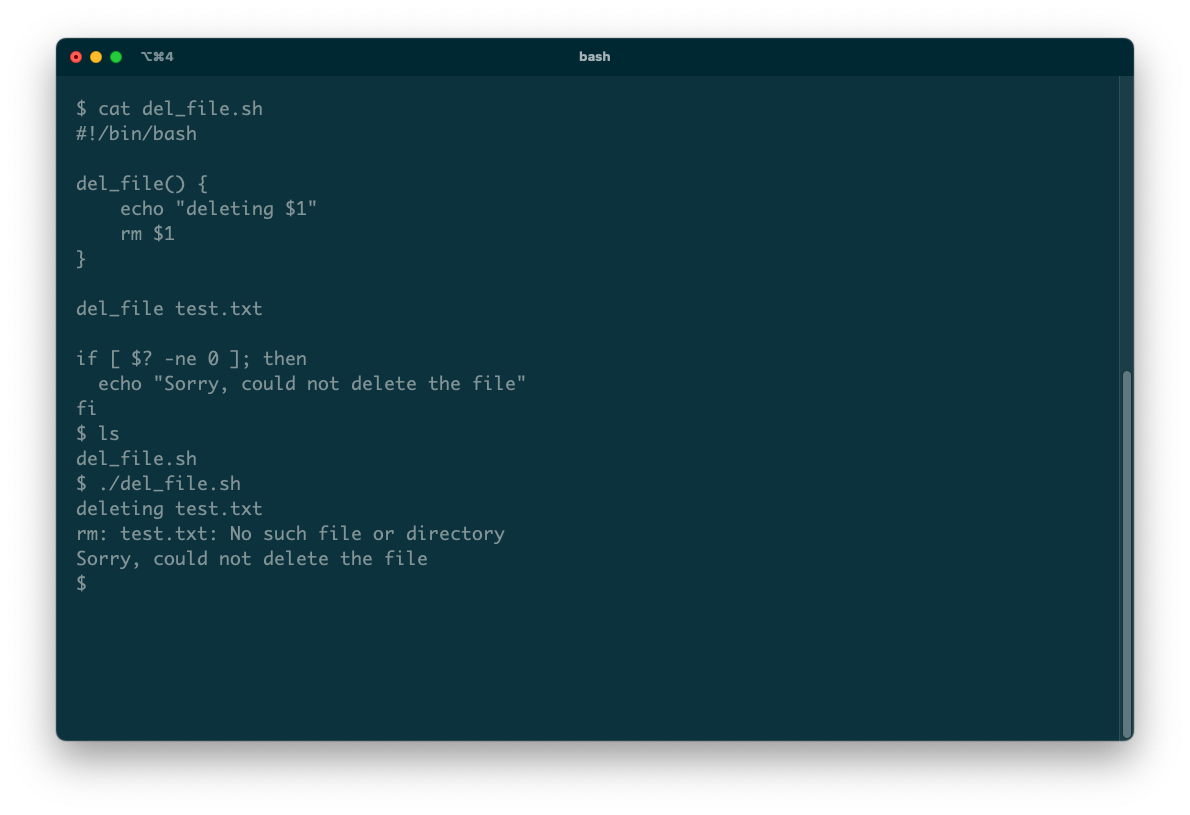
5. Create a Basic Calculator for Arithmetic Calculations
This final example demonstrates a very basic calculator. When you run it, you’ll enter two values, then choose an arithmetic operation to carry out on them.
Here’s the code for calc.sh:
#!/bin/bash
# Take operands as input
echo "Enter first number: "
read a
echo "Enter second number: "
read b
# Input type of operation
echo "Enter Arithmetic Operation Choice :"
echo "1. Addition"
echo "2. Subtraction"
echo "3. Multiplication"
echo "4. Division"
read choice
# calculator operations
case $choice in
1)
result=`echo $a + $b | bc`
;;
2)
result=`echo $a - $b | bc`
;;
3)
result=`echo $a \* $b | bc`
;;
4)
result=`echo "scale=2; $a / $b" | bc`
;;
esac
echo "Result: $result"
Note the use of case … esac which is Bash’s equivalent of the switch statement from other languages. It lets you test a value—in this case, the choice variable—against several fixed values and run associated code.
This script uses the bc command to carry out each calculation.
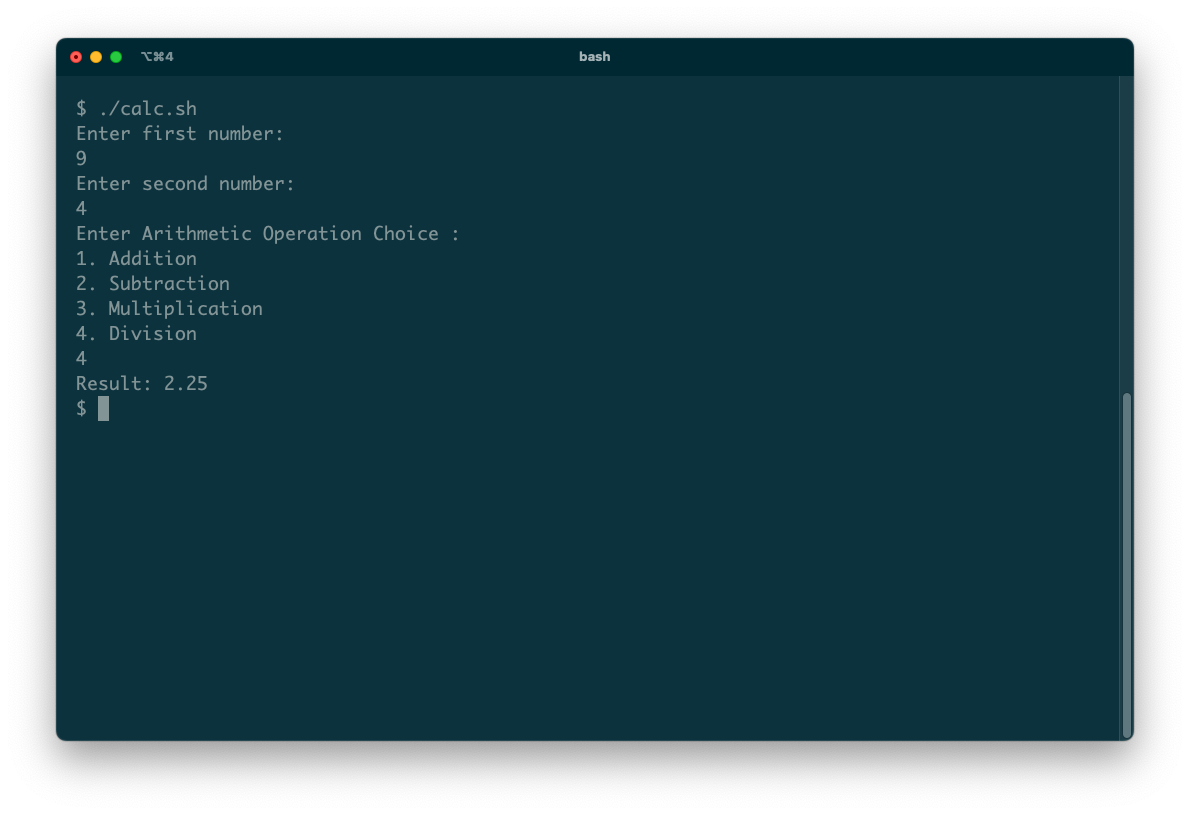